timeseries¶
Module: timeseries
¶
Inheritance diagram for nitime.timeseries
:
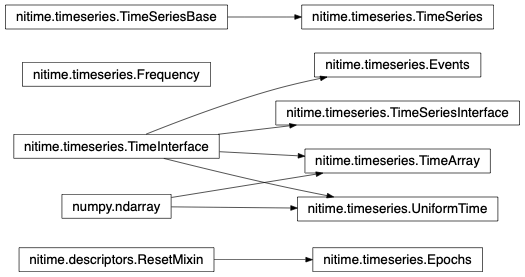
Base classes for generic time series analysis.
The classes implemented here are meant to provide fairly basic objects for managing time series data. They should serve mainly as data containers, with only minimal algorithmic functionality.
In the timeseries subpackage, there is a separate library of algorithms, and the classes defined here mostly delegate any computational facilities they may have to that library.
Over time, it is OK to add increasingly functionally rich classes, but only after their design is well proven in real-world use.
Classes¶
Epochs
¶
-
class
nitime.timeseries.
Epochs
(t0=None, stop=None, offset=None, start=None, duration=None, time_unit=None, static=None, **kwargs)¶ Bases:
nitime.descriptors.ResetMixin
Represents a time interval
-
__init__
(t0=None, stop=None, offset=None, start=None, duration=None, time_unit=None, static=None, **kwargs)¶ Parameters: t0 : 1-d array or TimeArray
A time relative to which the epochs started. Per default t0 and
- start are the same, but setting the offset parameter can adjust
that, so that the start-times are at a fixed time, relative to t0.
stop : 1-d array or TimeArray
The times of ends of epochs
offset : float, int or singleton TimeArray
A constant offset applied to t0 to set the starts of Epochs
start : 1-d array or TimeArray
The times of beginnings of epochs
duration : 1-d array or TimeArray
The durations of intervals.
time_unit : str, optional
The time unit of the object and all time-related things in it. Default: ‘s’
static : dict, optional
For fast initialization of an Epochs object from another Epochs object, this dict should contain all necessary items to have an Epoch defined.
-
duration
()¶ Duration array for the epoch
-
start
¶
-
stop
¶
-
Events
¶
-
class
nitime.timeseries.
Events
(time, labels=None, indices=None, time_unit=None, **data)¶ Bases:
nitime.timeseries.TimeInterface
Represents timestamps and associated data
-
__init__
(time, labels=None, indices=None, time_unit=None, **data)¶ Parameters: time : array or TimeArray
The times at which events occurred
labels : array, optional
indices : int array, optional
-
Frequency
¶
-
class
nitime.timeseries.
Frequency
¶ Bases:
float
A class for representation of the frequency (in Hz)
-
__init__
()¶ Initialize self. See help(type(self)) for accurate signature.
-
to_period
(time_unit='ps')¶ Convert the value of a frequency to the corresponding period (defaulting to a representation in the base_unit)
-
TimeArray
¶
-
class
nitime.timeseries.
TimeArray
¶ Bases:
numpy.ndarray
,nitime.timeseries.TimeInterface
Base-class for time representations, implementing the TimeInterface
-
__init__
()¶ Initialize self. See help(type(self)) for accurate signature.
-
at
(t, tol=None)¶ Returns the values of the TimeArray object at time t
-
convert_unit
(time_unit)¶ Convert from one time unit to another in place
-
during
(e)¶ Returns the values of the TimeArray object during Epoch e
-
index_at
(t, tol=None, mode='closest')¶ Returns the integer indices that corresponds to the time t
The returned indices depend on both tol and mode. The tol parameter specifies how close the given time must be to those present in the array to give a match, when mode is closest. The default tolerance is 1 base_unit (by default, picoseconds). If you specify the tolerance as 0, then only exact matches are allowed, be careful in this case of possible problems due to floating point roundoff error in your time specification.
When mode is before or after, the tolerance is completely ignored. In this case, either the largest time equal or before the given t or the earliest time equal or after the given t is returned.
Parameters: t : time-like
Anything that is valid input for a TimeArray constructor.
tol : time-like, optional
Tolerance, specified in the time units of this TimeArray.
mode : string
One of [‘closest’, ‘before’, ‘after’].
Returns: idx : The array with all the indices where the condition is met.
-
max
(axis=None, out=None)¶ Returns the maximal time
-
mean
(axis=None, dtype=None, out=None, keepdims=False)¶ Returns the average of the array elements along given axis.
Refer to numpy.mean for full documentation.
See also
numpy.mean
- equivalent function
-
min
(axis=None, out=None, keepdims=False)¶ Return the minimum along a given axis.
Refer to numpy.amin for full documentation.
See also
numpy.amin
- equivalent function
-
prod
(axis=None, dtype=None, out=None, keepdims=False)¶ Return the product of the array elements over the given axis
Refer to numpy.prod for full documentation.
See also
numpy.prod
- equivalent function
-
ptp
(axis=None, out=None, keepdims=False)¶ Peak to peak (maximum - minimum) value along a given axis.
Refer to numpy.ptp for full documentation.
See also
numpy.ptp
- equivalent function
-
slice_during
(e)¶ Returns the slice that corresponds to Epoch e
-
std
(*args, **kwargs)¶ Returns the standard deviation of this TimeArray (with time units) for detailed information, see numpy.std()
-
sum
(axis=None, dtype=None, out=None, keepdims=False)¶ Return the sum of the array elements over the given axis.
Refer to numpy.sum for full documentation.
See also
numpy.sum
- equivalent function
-
var
(axis=None, dtype=None, out=None, ddof=0, keepdims=False)¶ Returns the variance of the array elements, along given axis.
Refer to numpy.var for full documentation.
See also
numpy.var
- equivalent function
-
TimeSeries
¶
-
class
nitime.timeseries.
TimeSeries
(data, t0=None, sampling_interval=None, sampling_rate=None, duration=None, time=None, time_unit='s', metadata=None)¶ Bases:
nitime.timeseries.TimeSeriesBase
Represent data collected at uniform intervals.
-
__init__
(data, t0=None, sampling_interval=None, sampling_rate=None, duration=None, time=None, time_unit='s', metadata=None)¶ Create a new TimeSeries.
This class assumes that data is uniformly sampled, but you can specify the sampling in one of three (mutually exclusive) ways:
- sampling_interval [, t0]: data sampled starting at t0, equal intervals of sampling_interval.
- sampling_rate [, t0]: data sampled starting at t0, equal intervals of width 1/sampling_rate.
- time: a UniformTime object, in which case the TimeSeries can ‘inherit’ the properties of this object.
Parameters: data : array_like
Data array, interpreted as having its last dimension being time.
sampling_interval : float
Interval between successive time points.
sampling_rate : float
Inverse of the interval between successive time points.
t0 : float
If you provide a sampling rate, you can optionally also provide a starting time.
time :
Instead of sampling rate, you can explicitly provide an object of class UniformTime. Note that you can still also provide a different sampling_rate/sampling_interval/duration to take the place of the one in this object, but only as long as the changes are consistent with the length of the data.
time_unit : string
The unit of time.
Examples
The minimal specification of data and sampling interval:
>>> ts = TimeSeries([1,2,3],sampling_interval=0.25) >>> ts.time UniformTime([ 0. , 0.25, 0.5 ], time_unit='s') >>> ts.t0 0.0 s >>> ts.sampling_rate 4.0 Hz
Or data and sampling rate:
>>> ts = TimeSeries([1,2,3],sampling_rate=2) >>> ts.time UniformTime([ 0. , 0.5, 1. ], time_unit='s') >>> ts.t0 0.0 s >>> ts.sampling_interval 0.5 s
A time series where we specify the start time and sampling interval:
>>> ts = TimeSeries([1,2,3],t0=4.25,sampling_interval=0.5) >>> ts.data array([1, 2, 3]) >>> ts.time UniformTime([ 4.25, 4.75, 5.25], time_unit='s') >>> ts.t0 4.25 s >>> ts.sampling_interval 0.5 s >>> ts.sampling_rate 2.0 Hz
>>> ts = TimeSeries([1,2,3],t0=4.25,sampling_rate=2.0) >>> ts.data array([1, 2, 3]) >>> ts.time UniformTime([ 4.25, 4.75, 5.25], time_unit='s') >>> ts.t0 4.25 s >>> ts.sampling_interval 0.5 s >>> ts.sampling_rate 2.0 Hz
-
at
(t, tol=None)¶ Returns the values of the TimeArray object at time t
-
copy
()¶
-
during
(e)¶ Returns the TimeSeries slice corresponding to epoch e
-
static
from_time_and_data
(time, data)¶
-
shape
¶
-
time
()¶ Construct time array for the time-series object. This holds a UniformTime object, with properties derived from the TimeSeries object
-
TimeSeriesInterface
¶
-
class
nitime.timeseries.
TimeSeriesInterface
¶ Bases:
nitime.timeseries.TimeInterface
The minimally agreed upon interface for all time series.
This should be thought of as an abstract base class.
-
__init__
()¶ Initialize self. See help(type(self)) for accurate signature.
-
data
= None¶
-
metadata
= None¶
-
time
= None¶
-
UniformTime
¶
-
class
nitime.timeseries.
UniformTime
¶ Bases:
numpy.ndarray
,nitime.timeseries.TimeInterface
A representation of time sampled uniformly
-
__init__
()¶ Initialize self. See help(type(self)) for accurate signature.
-
at
(t)¶ Returns the values of the UniformTime object at time t
-
during
(e)¶ Returns the values of the UniformTime object during Epoch e
-
index_at
(t, boolean=False)¶ Find the index that corresponds to the time bin containing t
Returns boolean mask if boolean=True and integer indices otherwise.
-
max
(axis=None, out=None)¶ Returns the maximal time
-
min
(axis=None, out=None)¶ Returns the minimal time
-
slice_during
(e)¶ Returns the slice that corresponds to Epoch e
-
Functions¶
-
nitime.timeseries.
concatenate_time_series
(time_series_seq)¶ Concatenates a sequence of time-series objects in time.
The input can be any iterable of time-series objects; metadata, sampling rates and other attributes are kept from the last one in the sequence.
This one requires that all the time-series in the list have the same sampling rate and that all the data have the same number of items in all dimensions, except the time dimension
-
nitime.timeseries.
get_time_unit
(obj)¶ Extract the time unit of the object. If it is an iterable, get the time unit of the first element.
-
nitime.timeseries.
str_tspec
(tspec, arg_names)¶ Turn a single tspec into human readable form
-
nitime.timeseries.
str_valid_tspecs
(valid_tspecs, arg_names)¶ Given a set of valid_tspecs, return a string that turns them into human-readable form