algorithms.registration.groupwise_registration¶
Module: algorithms.registration.groupwise_registration
¶
Inheritance diagram for nipy.algorithms.registration.groupwise_registration
:
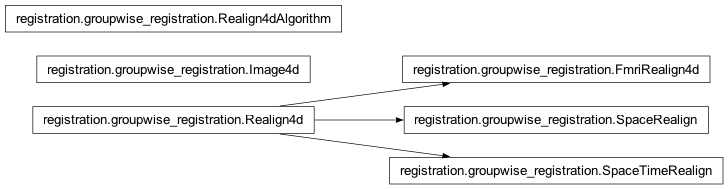
Motion correction / motion correction with slice timing
Routines implementing motion correction and motion correction combined with slice-timing.
See:
Roche, Alexis (2011) A four-dimensional registration algorithm with application to joint correction of motion and slice timing in fMRI. Medical Imaging, IEEE Transactions on; 30:1546–1554
Classes¶
FmriRealign4d
¶
- class nipy.algorithms.registration.groupwise_registration.FmriRealign4d(images, slice_order=None, tr=None, tr_slices=None, start=0.0, interleaved=None, time_interp=None, slice_times=None, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>, slice_info=None)¶
Bases:
Realign4d
- __init__(images, slice_order=None, tr=None, tr_slices=None, start=0.0, interleaved=None, time_interp=None, slice_times=None, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>, slice_info=None)¶
Spatiotemporal realignment class for fMRI series. This class is similar to Realign4d but provides a more flexible API for initialization in order to make it easier to declare slice acquisition times for standard sequences.
Warning: this class is deprecated; please use
SpaceTimeRealign
instead.- Parameters:
- imagesimage or list of images
Single or multiple input 4d images representing one or several fMRI runs.
- slice_orderstr or array-like
If str, one of {‘ascending’, ‘descending’}. If array-like, then the order in which the slices were collected in time. For instance, the following represents an ascending contiguous sequence:
slice_order = [0, 1, 2, …]
Note that slice_order differs from the argument used e.g. in the SPM slice timing routine in that it maps spatial slice positions to slice times. It is a mapping from space to time, while SPM conventionally uses the reverse mapping from time to space. For example, for an interleaved sequence with 10 slices, where we acquired slice 0 (in space) first, then slice 2 (in space) etc, slice_order would be [0, 5, 1, 6, 2, 7, 3, 8, 4, 9]
Using slice_order assumes that the inter-slice acquisition time is constant throughout acquisition. If this is not the case, use the slice_times argument instead and leave slice_order to None.
- trfloat
Inter-scan repetition time, i.e. the time elapsed between two consecutive scans. The unit in which tr is given is arbitrary although it needs to be consistent with the tr_slices and start arguments if provided. If None, tr is computed internally assuming a regular slice acquisition scheme.
- tr_slicesfloat
Inter-slice repetition time, same as tr for slices. If None, acquisition is assumed regular and tr_slices is set to tr divided by the number of slices.
- startfloat
Starting acquisition time (time of the first acquired slice) respective to the time origin for resampling. start is assumed to be given in the same unit as tr. Setting start=0 means that the resampled data will be synchronous with the first acquired slice. Setting start=-tr/2 means that the resampled data will be synchronous with the slice acquired at half repetition time.
- time_interp: bool
Tells whether time interpolation is used or not within the realignment algorithm. If False, slices are considered to be acquired all at the same time, thus no slice timing correction will be performed.
- interleavedbool
Deprecated argument.
Tells whether slice acquisition order is interleaved in a certain sense. Setting interleaved to True or False will trigger an error unless slice_order is ‘ascending’ or ‘descending’ and slice_times is None.
If slice_order==’ascending’ and interleaved==True, the assumed slice order is (assuming 10 slices):
[0, 5, 1, 6, 2, 7, 3, 8, 4, 9]
If slice_order==’descending’ and interleaved==True, the assumed slice order is:
[9, 4, 8, 3, 7, 2, 6, 1, 5, 0]
WARNING: given that there exist other types of interleaved acquisitions depending on scanner settings and manufacturers, you should refrain from using the interleaved keyword argument unless you are sure what you are doing. It is generally safer to explicitly input slice_order or slice_times.
- slice_timesNone, str or array-like
This argument can be used instead of slice_order, tr_slices, start and time_interp altogether.
If None, slices are assumed to be acquired simultaneously hence no slice timing correction is performed. If array-like, then slice_times gives the slice acquisition times along the slice axis in units that are consistent with the provided tr.
Generally speaking, the following holds for sequences with constant inter-slice repetition time tr_slices:
slice_times = start + tr_slices * slice_order
For other sequences such as, e.g., sequences with simultaneously acquired slices, it is necessary to input slice_times explicitly along with tr.
- slice_infoNone or tuple, optional
None, or a tuple with slice axis as the first element and direction as the second, for instance (2, 1). If None, then the slice axis and direction are guessed from the first run’s affine assuming that slices are collected along the closest axis to the z-axis. This means that we assume by default an axial acquisition with slice axis pointing from bottom to top of the head.
- estimate(loops=5, between_loops=None, align_runs=True, speedup=5, refscan=0, borders=(1, 1, 1), optimizer='ncg', xtol=1e-05, ftol=1e-05, gtol=1e-05, stepsize=1e-06, maxiter=64, maxfun=None)¶
Estimate motion parameters.
- Parameters:
- loopsint or sequence of ints
Determines the number of iterations performed to realign scans within each run for each pass defined by the
speedup
argument. For instance, settingspeedup
== (5,2) andloops
== (5,1) means that 5 iterations are performed in a first pass where scans are subsampled by an isotropic factor 5, followed by one iteration where scans are subsampled by a factor 2.- between_loopsNone, int or sequence of ints
Similar to
loops
for between-run motion estimation. Determines the number of iterations used to realign scans across runs, a procedure similar to within-run realignment that uses the mean images from each run. If None, assumed to be the same asloops
. The setting used in the experiments described in Roche, IEEE TMI 2011, was:speedup
= (5, 2),loops
= (5, 1) andbetween_loops
= (5, 1).- align_runsbool
Determines whether between-run motion is estimated or not. If False, the
between_loops
argument is ignored.- speedup: int or sequence of ints
Determines an isotropic sub-sampling factor, or a sequence of such factors, applied to the scans to perform motion estimation. If a sequence, several estimation passes are applied.
- refscanNone or int
Defines the number of the scan used as the reference coordinate system for each run. If None, a reference coordinate system is defined internally that does not correspond to any particular scan. Note that the coordinate system associated with the first run is always
- borderssequence of ints
Should be of length 3. Determines the field of view for motion estimation in terms of the number of slices at each extremity of the reference grid that are ignored for motion parameter estimation. For instance,
borders``==(1,1,1) means that the realignment cost function will not take into account voxels located in the first and last axial/sagittal/coronal slices in the reference grid. Please note that this choice only affects parameter estimation but does not affect image resampling in any way, see ``resample
method.- optimizerstr
Defines the optimization method. One of ‘simplex’, ‘powell’, ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- xtolfloat
Tolerance on variations of transformation parameters to test numerical convergence.
- ftolfloat
Tolerance on variations of the intensity comparison metric to test numerical convergence.
- gtolfloat
Tolerance on the gradient of the intensity comparison metric to test numerical convergence. Applicable to optimizers ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- stepsizefloat
Step size to approximate the gradient and Hessian of the intensity comparison metric w.r.t. transformation parameters. Applicable to optimizers ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- maxiterint
Maximum number of iterations in optimization.
- maxfunint
Maximum number of function evaluations in maxfun.
- resample(r=None, align_runs=True)¶
Return the resampled run number r as a 4d nipy-like image. Returns all runs as a list of images if r is None.
Image4d
¶
- class nipy.algorithms.registration.groupwise_registration.Image4d(data, affine, tr, slice_times, slice_info=None)¶
Bases:
object
Class to represent a sequence of 3d scans (possibly acquired on a slice-by-slice basis).
Object remains empty until the data array is actually loaded in memory.
- Parameters:
- datand array or proxy (function that actually gets the array)
- __init__(data, affine, tr, slice_times, slice_info=None)¶
Configure fMRI acquisition time parameters.
- free_data()¶
- get_fdata()¶
- get_shape()¶
- scanner_time(zv, t)¶
tv = scanner_time(zv, t) zv, tv are grid coordinates; t is an actual time value.
- z_to_slice(z)¶
Account for the fact that slices may be stored in reverse order wrt the scanner coordinate system convention (slice 0 == bottom of the head)
Realign4d
¶
- class nipy.algorithms.registration.groupwise_registration.Realign4d(images, tr, slice_times=None, slice_info=None, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>)¶
Bases:
object
- __init__(images, tr, slice_times=None, slice_info=None, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>)¶
Spatiotemporal realignment class for series of 3D images.
The algorithm performs simultaneous motion and slice timing correction for fMRI series or other data where slices are not acquired simultaneously.
- Parameters:
- imagesimage or list of images
Single or multiple input 4d images representing one or several sessions.
- trfloat
Inter-scan repetition time, i.e. the time elapsed between two consecutive scans. The unit in which tr is given is arbitrary although it needs to be consistent with the slice_times argument.
- slice_timesNone or array-like
If None, slices are assumed to be acquired simultaneously hence no slice timing correction is performed. If array-like, then the slice acquisition times.
- slice_infoNone or tuple, optional
None, or a tuple with slice axis as the first element and direction as the second, for instance (2, 1). If None, then guess the slice axis, and direction, as the closest to the z axis, as estimated from the affine.
- estimate(loops=5, between_loops=None, align_runs=True, speedup=5, refscan=0, borders=(1, 1, 1), optimizer='ncg', xtol=1e-05, ftol=1e-05, gtol=1e-05, stepsize=1e-06, maxiter=64, maxfun=None)¶
Estimate motion parameters.
- Parameters:
- loopsint or sequence of ints
Determines the number of iterations performed to realign scans within each run for each pass defined by the
speedup
argument. For instance, settingspeedup
== (5,2) andloops
== (5,1) means that 5 iterations are performed in a first pass where scans are subsampled by an isotropic factor 5, followed by one iteration where scans are subsampled by a factor 2.- between_loopsNone, int or sequence of ints
Similar to
loops
for between-run motion estimation. Determines the number of iterations used to realign scans across runs, a procedure similar to within-run realignment that uses the mean images from each run. If None, assumed to be the same asloops
. The setting used in the experiments described in Roche, IEEE TMI 2011, was:speedup
= (5, 2),loops
= (5, 1) andbetween_loops
= (5, 1).- align_runsbool
Determines whether between-run motion is estimated or not. If False, the
between_loops
argument is ignored.- speedup: int or sequence of ints
Determines an isotropic sub-sampling factor, or a sequence of such factors, applied to the scans to perform motion estimation. If a sequence, several estimation passes are applied.
- refscanNone or int
Defines the number of the scan used as the reference coordinate system for each run. If None, a reference coordinate system is defined internally that does not correspond to any particular scan. Note that the coordinate system associated with the first run is always
- borderssequence of ints
Should be of length 3. Determines the field of view for motion estimation in terms of the number of slices at each extremity of the reference grid that are ignored for motion parameter estimation. For instance,
borders``==(1,1,1) means that the realignment cost function will not take into account voxels located in the first and last axial/sagittal/coronal slices in the reference grid. Please note that this choice only affects parameter estimation but does not affect image resampling in any way, see ``resample
method.- optimizerstr
Defines the optimization method. One of ‘simplex’, ‘powell’, ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- xtolfloat
Tolerance on variations of transformation parameters to test numerical convergence.
- ftolfloat
Tolerance on variations of the intensity comparison metric to test numerical convergence.
- gtolfloat
Tolerance on the gradient of the intensity comparison metric to test numerical convergence. Applicable to optimizers ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- stepsizefloat
Step size to approximate the gradient and Hessian of the intensity comparison metric w.r.t. transformation parameters. Applicable to optimizers ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- maxiterint
Maximum number of iterations in optimization.
- maxfunint
Maximum number of function evaluations in maxfun.
- resample(r=None, align_runs=True)¶
Return the resampled run number r as a 4d nipy-like image. Returns all runs as a list of images if r is None.
Realign4dAlgorithm
¶
- class nipy.algorithms.registration.groupwise_registration.Realign4dAlgorithm(im4d, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>, transforms=None, time_interp=True, subsampling=(1, 1, 1), refscan=0, borders=(1, 1, 1), optimizer='ncg', optimize_template=True, xtol=1e-05, ftol=1e-05, gtol=1e-05, stepsize=1e-06, maxiter=64, maxfun=None)¶
Bases:
object
- __init__(im4d, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>, transforms=None, time_interp=True, subsampling=(1, 1, 1), refscan=0, borders=(1, 1, 1), optimizer='ncg', optimize_template=True, xtol=1e-05, ftol=1e-05, gtol=1e-05, stepsize=1e-06, maxiter=64, maxfun=None)¶
- align_to_refscan()¶
The motion_estimate method aligns scans with an online template so that spatial transforms map some average head space to the scanner space. To conventionally redefine the head space as being aligned with some reference scan, we need to right compose each head_average-to-scanner transform with the refscan’s ‘to head_average’ transform.
- estimate_instant_motion(t)¶
Estimate motion parameters at a particular time.
- estimate_motion()¶
Optimize motion parameters for the whole sequence. All the time frames are initially resampled according to the current space/time transformation, the parameters of which are further optimized sequentially.
- init_instant_motion(t)¶
Pre-compute and cache some constants (at fixed time) for repeated computations of the alignment energy.
The idea is to decompose the average temporal variance via:
V = (n-1)/n V* + (n-1)/n^2 (x-m*)^2
with x the considered volume at time t, and m* the mean of all resampled volumes but x. Only the second term is variable when
one volumes while the others are fixed. A similar decomposition is used for the global variance, so we end up with:
V/V0 = [nV* + (x-m*)^2] / [nV0* + (x-m0*)^2]
- resample(t)¶
Resample a particular time frame on the (sub-sampled) working grid.
x,y,z,t are “head” grid coordinates X,Y,Z,T are “scanner” grid coordinates
- resample_full_data()¶
- set_fmin(optimizer, stepsize, **kwargs)¶
Return the minimization function
- set_transform(t, pc)¶
SpaceRealign
¶
- class nipy.algorithms.registration.groupwise_registration.SpaceRealign(images, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>)¶
Bases:
Realign4d
- __init__(images, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>)¶
Spatial registration of time series with no time interpolation
- Parameters:
- imagesimage or list of images
Single or multiple input 4d images representing one or several fMRI runs.
- affine_class
Affine
class, optional transformation class to use to calculate transformations between the volumes. Default is :class:
Rigid
- estimate(loops=5, between_loops=None, align_runs=True, speedup=5, refscan=0, borders=(1, 1, 1), optimizer='ncg', xtol=1e-05, ftol=1e-05, gtol=1e-05, stepsize=1e-06, maxiter=64, maxfun=None)¶
Estimate motion parameters.
- Parameters:
- loopsint or sequence of ints
Determines the number of iterations performed to realign scans within each run for each pass defined by the
speedup
argument. For instance, settingspeedup
== (5,2) andloops
== (5,1) means that 5 iterations are performed in a first pass where scans are subsampled by an isotropic factor 5, followed by one iteration where scans are subsampled by a factor 2.- between_loopsNone, int or sequence of ints
Similar to
loops
for between-run motion estimation. Determines the number of iterations used to realign scans across runs, a procedure similar to within-run realignment that uses the mean images from each run. If None, assumed to be the same asloops
. The setting used in the experiments described in Roche, IEEE TMI 2011, was:speedup
= (5, 2),loops
= (5, 1) andbetween_loops
= (5, 1).- align_runsbool
Determines whether between-run motion is estimated or not. If False, the
between_loops
argument is ignored.- speedup: int or sequence of ints
Determines an isotropic sub-sampling factor, or a sequence of such factors, applied to the scans to perform motion estimation. If a sequence, several estimation passes are applied.
- refscanNone or int
Defines the number of the scan used as the reference coordinate system for each run. If None, a reference coordinate system is defined internally that does not correspond to any particular scan. Note that the coordinate system associated with the first run is always
- borderssequence of ints
Should be of length 3. Determines the field of view for motion estimation in terms of the number of slices at each extremity of the reference grid that are ignored for motion parameter estimation. For instance,
borders``==(1,1,1) means that the realignment cost function will not take into account voxels located in the first and last axial/sagittal/coronal slices in the reference grid. Please note that this choice only affects parameter estimation but does not affect image resampling in any way, see ``resample
method.- optimizerstr
Defines the optimization method. One of ‘simplex’, ‘powell’, ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- xtolfloat
Tolerance on variations of transformation parameters to test numerical convergence.
- ftolfloat
Tolerance on variations of the intensity comparison metric to test numerical convergence.
- gtolfloat
Tolerance on the gradient of the intensity comparison metric to test numerical convergence. Applicable to optimizers ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- stepsizefloat
Step size to approximate the gradient and Hessian of the intensity comparison metric w.r.t. transformation parameters. Applicable to optimizers ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- maxiterint
Maximum number of iterations in optimization.
- maxfunint
Maximum number of function evaluations in maxfun.
- resample(r=None, align_runs=True)¶
Return the resampled run number r as a 4d nipy-like image. Returns all runs as a list of images if r is None.
SpaceTimeRealign
¶
- class nipy.algorithms.registration.groupwise_registration.SpaceTimeRealign(images, tr, slice_times, slice_info, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>)¶
Bases:
Realign4d
- __init__(images, tr, slice_times, slice_info, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>)¶
Spatiotemporal realignment class for fMRI series.
This class gives a high-level interface to
Realign4d
- Parameters:
- imagesimage or list of images
Single or multiple input 4d images representing one or several fMRI runs.
- trNone or float or “header-allow-1.0”
Inter-scan repetition time in seconds, i.e. the time elapsed between two consecutive scans. If None, an attempt is made to read the TR from the header, but an exception is thrown for values 0 or 1. A value of “header-allow-1.0” will signal to accept a header TR of 1.
- slice_timesstr or callable or array-like
If str, one of the function names in
SLICETIME_FUNCTIONS
dictionary fromnipy.algorithms.slicetiming.timefuncs
. If callable, a function taking two parameters:n_slices
andtr
(number of slices in the images, inter-scan repetition time in seconds). This function returns a vector of times of slice acquisition \(t_i\) for each slice \(i\) in the volumes. Seenipy.algorithms.slicetiming.timefuncs
for a collection of functions for common slice acquisition schemes. If array-like, then should be a slice time vector as above.- slice_infoint or length 2 sequence
If int, the axis in images that is the slice axis. In a 4D image, this will often be axis = 2. If a 2 sequence, then elements are
(slice_axis, slice_direction)
, whereslice_axis
is the slice axis in the image as above, andslice_direction
is 1 if the slices were acquired slice 0 first, slice -1 last, or -1 if acquired slice -1 first, slice 0 last. If slice_info is an int, assumeslice_direction
== 1.- affine_class
Affine
class, optional transformation class to use to calculate transformations between the volumes. Default is :class:
Rigid
- estimate(loops=5, between_loops=None, align_runs=True, speedup=5, refscan=0, borders=(1, 1, 1), optimizer='ncg', xtol=1e-05, ftol=1e-05, gtol=1e-05, stepsize=1e-06, maxiter=64, maxfun=None)¶
Estimate motion parameters.
- Parameters:
- loopsint or sequence of ints
Determines the number of iterations performed to realign scans within each run for each pass defined by the
speedup
argument. For instance, settingspeedup
== (5,2) andloops
== (5,1) means that 5 iterations are performed in a first pass where scans are subsampled by an isotropic factor 5, followed by one iteration where scans are subsampled by a factor 2.- between_loopsNone, int or sequence of ints
Similar to
loops
for between-run motion estimation. Determines the number of iterations used to realign scans across runs, a procedure similar to within-run realignment that uses the mean images from each run. If None, assumed to be the same asloops
. The setting used in the experiments described in Roche, IEEE TMI 2011, was:speedup
= (5, 2),loops
= (5, 1) andbetween_loops
= (5, 1).- align_runsbool
Determines whether between-run motion is estimated or not. If False, the
between_loops
argument is ignored.- speedup: int or sequence of ints
Determines an isotropic sub-sampling factor, or a sequence of such factors, applied to the scans to perform motion estimation. If a sequence, several estimation passes are applied.
- refscanNone or int
Defines the number of the scan used as the reference coordinate system for each run. If None, a reference coordinate system is defined internally that does not correspond to any particular scan. Note that the coordinate system associated with the first run is always
- borderssequence of ints
Should be of length 3. Determines the field of view for motion estimation in terms of the number of slices at each extremity of the reference grid that are ignored for motion parameter estimation. For instance,
borders``==(1,1,1) means that the realignment cost function will not take into account voxels located in the first and last axial/sagittal/coronal slices in the reference grid. Please note that this choice only affects parameter estimation but does not affect image resampling in any way, see ``resample
method.- optimizerstr
Defines the optimization method. One of ‘simplex’, ‘powell’, ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- xtolfloat
Tolerance on variations of transformation parameters to test numerical convergence.
- ftolfloat
Tolerance on variations of the intensity comparison metric to test numerical convergence.
- gtolfloat
Tolerance on the gradient of the intensity comparison metric to test numerical convergence. Applicable to optimizers ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- stepsizefloat
Step size to approximate the gradient and Hessian of the intensity comparison metric w.r.t. transformation parameters. Applicable to optimizers ‘cg’, ‘ncg’, ‘bfgs’ and ‘steepest’.
- maxiterint
Maximum number of iterations in optimization.
- maxfunint
Maximum number of function evaluations in maxfun.
- resample(r=None, align_runs=True)¶
Return the resampled run number r as a 4d nipy-like image. Returns all runs as a list of images if r is None.
Functions¶
- nipy.algorithms.registration.groupwise_registration.adjust_subsampling(speedup, dims)¶
- nipy.algorithms.registration.groupwise_registration.guess_slice_axis_and_direction(slice_info, affine)¶
- nipy.algorithms.registration.groupwise_registration.interp_slice_times(Z, slice_times, tr)¶
- nipy.algorithms.registration.groupwise_registration.make_grid(dims, subsampling=(1, 1, 1), borders=(0, 0, 0))¶
- nipy.algorithms.registration.groupwise_registration.realign4d(runs, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>, time_interp=True, align_runs=True, loops=5, between_loops=5, speedup=5, refscan=0, borders=(1, 1, 1), optimizer='ncg', xtol=1e-05, ftol=1e-05, gtol=1e-05, stepsize=1e-06, maxiter=64, maxfun=None)¶
- Parameters:
- runslist of Image4d objects
- Returns:
- transformslist
nested list of rigid transformations
- transforms map an ‘ideal’ 4d grid (conventionally aligned with the
- first scan of the first run) to the ‘acquisition’ 4d grid for each
- run
- nipy.algorithms.registration.groupwise_registration.resample4d(im4d, transforms, time_interp=True)¶
Resample a 4D image according to the specified sequence of spatial transforms, using either 4D interpolation if time_interp is True and 3D interpolation otherwise.
- nipy.algorithms.registration.groupwise_registration.scanner_coords(xyz, affine, from_world, to_world)¶
- nipy.algorithms.registration.groupwise_registration.single_run_realign4d(im4d, affine_class=<class 'nipy.algorithms.registration.affine.Rigid'>, time_interp=True, loops=5, speedup=5, refscan=0, borders=(1, 1, 1), optimizer='ncg', xtol=1e-05, ftol=1e-05, gtol=1e-05, stepsize=1e-06, maxiter=64, maxfun=None)¶
Realign a single run in space and time.
- Parameters:
- im4dImage4d instance
- speedupint or sequence
If a sequence, implement a multi-scale realignment
- nipy.algorithms.registration.groupwise_registration.tr_from_header(images)¶
Return the TR from the header of an image or list of images.
- Parameters:
- imagesimage or list of images
Single or multiple input 4d images representing one or several sessions.
- Returns:
- float
Repetition time, as specified in NIfTI header.
- Raises:
- ValueError
if the TR between the images is inconsistent.