core.reference.spaces¶
Module: core.reference.spaces
¶
Inheritance diagram for nipy.core.reference.spaces
:
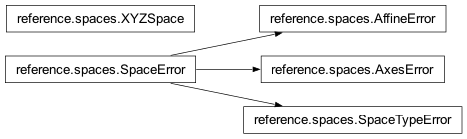
Useful neuroimaging coordinate map makers and utilities
Classes¶
AffineError
¶
- class nipy.core.reference.spaces.AffineError¶
Bases:
SpaceError
- __init__(*args, **kwargs)¶
- args¶
- with_traceback()¶
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
AxesError
¶
- class nipy.core.reference.spaces.AxesError¶
Bases:
SpaceError
- __init__(*args, **kwargs)¶
- args¶
- with_traceback()¶
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
SpaceError
¶
SpaceTypeError
¶
- class nipy.core.reference.spaces.SpaceTypeError¶
Bases:
SpaceError
- __init__(*args, **kwargs)¶
- args¶
- with_traceback()¶
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
XYZSpace
¶
- class nipy.core.reference.spaces.XYZSpace(name)¶
Bases:
object
Class contains logic for spaces with XYZ coordinate systems
>>> sp = XYZSpace('hijo') >>> print(sp) hijo: [('x', 'hijo-x=L->R'), ('y', 'hijo-y=P->A'), ('z', 'hijo-z=I->S')] >>> csm = sp.to_coordsys_maker() >>> cs = csm(3) >>> cs CoordinateSystem(coord_names=('hijo-x=L->R', 'hijo-y=P->A', 'hijo-z=I->S'), name='hijo', coord_dtype=float64) >>> cs in sp True
- __init__(name)¶
- as_map()¶
Return xyz names as dictionary
>>> sp = XYZSpace('hijo') >>> sorted(sp.as_map().items()) [('x', 'hijo-x=L->R'), ('y', 'hijo-y=P->A'), ('z', 'hijo-z=I->S')]
- as_tuple()¶
Return xyz names as tuple
>>> sp = XYZSpace('hijo') >>> sp.as_tuple() ('hijo-x=L->R', 'hijo-y=P->A', 'hijo-z=I->S')
- register_to(mapping)¶
Update mapping with key=self.x, value=’x’ etc pairs
The mapping will then have keys that are names we (
self
) identify as being x, or y, or z, values are ‘x’ or ‘y’ or ‘z’.Note that this is the opposite way round for keys, values, compared to the
as_map
method.- Parameters:
- mappingmapping
such as a dict
- Returns:
- None
Examples
>>> sp = XYZSpace('hijo') >>> mapping = {} >>> sp.register_to(mapping) >>> sorted(mapping.items()) [('hijo-x=L->R', 'x'), ('hijo-y=P->A', 'y'), ('hijo-z=I->S', 'z')]
- to_coordsys_maker(extras=())¶
Make a coordinate system maker for this space
- Parameters:
- extrasequence
names for any further axes after x, y, z
- Returns:
- csmCoordinateSystemMaker
Examples
>>> sp = XYZSpace('hijo') >>> csm = sp.to_coordsys_maker() >>> csm(3) CoordinateSystem(coord_names=('hijo-x=L->R', 'hijo-y=P->A', 'hijo-z=I->S'), name='hijo', coord_dtype=float64)
- property x¶
x-space coordinate name
- x_suffix = 'x=L->R'¶
- property y¶
y-space coordinate name
- y_suffix = 'y=P->A'¶
- property z¶
z-space coordinate name
- z_suffix = 'z=I->S'¶
Functions¶
- nipy.core.reference.spaces.get_world_cs(world_id, ndim=3, extras='tuvw', spaces=None)¶
Get world coordinate system from world_id
- Parameters:
- world_idstr, XYZSPace, CoordSysMaker or CoordinateSystem
Object defining a world output system. If str, then should be a name of an XYZSpace in the list spaces.
- ndimint, optional
Number of dimensions in this world. Default is 3
- extrassequence, optional
Coordinate (axis) names for axes > 3 that are not named by world_id
- spacesNone or sequence, optional
List of known (named) spaces to compare a str world_id to. If None, use the module level
known_spaces
- Returns:
- world_csCoordinateSystem
A world coordinate system
Examples
>>> get_world_cs('mni') CoordinateSystem(coord_names=('mni-x=L->R', 'mni-y=P->A', 'mni-z=I->S'), name='mni', coord_dtype=float64)
>>> get_world_cs(mni_space, 4) CoordinateSystem(coord_names=('mni-x=L->R', 'mni-y=P->A', 'mni-z=I->S', 't'), name='mni', coord_dtype=float64)
>>> from nipy.core.api import CoordinateSystem >>> get_world_cs(CoordinateSystem('xyz')) CoordinateSystem(coord_names=('x', 'y', 'z'), name='', coord_dtype=float64)
- nipy.core.reference.spaces.is_xyz_affable(coordmap, name2xyz=None)¶
Return True if the coordap has an xyz affine
- Parameters:
- coordmap
CoordinateMap
instance Coordinate map to test
- name2xyzNone or mapping, optional
Object such that
name2xyz[ax_name]
returns ‘x’, or ‘y’ or ‘z’ or raises a KeyError for a strax_name
. None means use module default.
- coordmap
- Returns:
- tfbool
True if coordmap has an xyz affine, False otherwise
Examples
>>> cmap = vox2mni(np.diag([2,3,4,5,1])) >>> cmap AffineTransform( function_domain=CoordinateSystem(coord_names=('i', 'j', 'k', 'l'), name='voxels', coord_dtype=float64), function_range=CoordinateSystem(coord_names=('mni-x=L->R', 'mni-y=P->A', 'mni-z=I->S', 't'), name='mni', coord_dtype=float64), affine=array([[ 2., 0., 0., 0., 0.], [ 0., 3., 0., 0., 0.], [ 0., 0., 4., 0., 0.], [ 0., 0., 0., 5., 0.], [ 0., 0., 0., 0., 1.]]) ) >>> is_xyz_affable(cmap) True >>> time0_cmap = cmap.reordered_domain([3,0,1,2]) >>> time0_cmap AffineTransform( function_domain=CoordinateSystem(coord_names=('l', 'i', 'j', 'k'), name='voxels', coord_dtype=float64), function_range=CoordinateSystem(coord_names=('mni-x=L->R', 'mni-y=P->A', 'mni-z=I->S', 't'), name='mni', coord_dtype=float64), affine=array([[ 0., 2., 0., 0., 0.], [ 0., 0., 3., 0., 0.], [ 0., 0., 0., 4., 0.], [ 5., 0., 0., 0., 0.], [ 0., 0., 0., 0., 1.]]) ) >>> is_xyz_affable(time0_cmap) False
- nipy.core.reference.spaces.is_xyz_space(obj)¶
True if obj appears to be an XYZ space definition
- nipy.core.reference.spaces.known_space(obj, spaces=None)¶
If obj is in a known space, return the space, otherwise return None
- Parameters:
- objobject
Object that can be tested against an XYZSpace with
obj in sp
- spacesNone or sequence, optional
spaces to test against. If None, use the module level
known_spaces
list to test against.
- Returns:
- spNone or XYZSpace
If obj is not in any of the known_spaces, return None. Otherwise return the first matching space in known_spaces
Examples
>>> from nipy.core.api import CoordinateSystem >>> sp0 = XYZSpace('hijo') >>> sp1 = XYZSpace('hija')
Make a matching coordinate system
>>> cs = sp0.to_coordsys_maker()(3)
Test whether this coordinate system is in either of
(sp0, sp1)
>>> known_space(cs, (sp0, sp1)) XYZSpace('hijo')
So, yes, it’s in
sp0
. How about another generic CoordinateSystem?>>> known_space(CoordinateSystem('xyz'), (sp0, sp1)) is None True
So, no, that is not in either of
(sp0, sp1)
- nipy.core.reference.spaces.xyz_affine(coordmap, name2xyz=None)¶
Return (4, 4) affine mapping voxel coordinates to XYZ from coordmap
If no (4, 4) affine “makes sense”(TM) for this coordmap then raise errors listed below. A (4, 4) affine makes sense if the first three output axes are recognizably X, Y, and Z in that order AND they there are corresponding input dimensions, AND the corresponding input dimensions are the first three input dimension (in any order). Thus the input axes have to be 3D.
- Parameters:
- coordmap
CoordinateMap
instance - name2xyzNone or mapping, optional
Object such that
name2xyz[ax_name]
returns ‘x’, or ‘y’ or ‘z’ or raises a KeyError for a strax_name
. None means use module default.
- coordmap
- Returns:
- xyz_aff(4,4) array
voxel to X, Y, Z affine mapping
- Raises:
- SpaceTypeErrorif this is not an affine coordinate map
- AxesErrorif not all of x, y, z recognized in coordmap output, or they
- are in the wrong order, or the x, y, z axes do not correspond to the first
- three input axes.
- AffineErrorif axes dropped from the affine contribute to x, y, z
- coordinates.
Notes
We could also try and “make sense” (TM) of a coordmap that had X, Y and Z outputs, but not in that order, nor all in the first three axes. In that case we could just permute the affine to get the output order we need. But, that could become confusing if the returned affine has different output coordinates than the passed coordmap. And it’s more complicated. So, let’s not do that for now.
Examples
>>> cmap = vox2mni(np.diag([2,3,4,5,1])) >>> cmap AffineTransform( function_domain=CoordinateSystem(coord_names=('i', 'j', 'k', 'l'), name='voxels', coord_dtype=float64), function_range=CoordinateSystem(coord_names=('mni-x=L->R', 'mni-y=P->A', 'mni-z=I->S', 't'), name='mni', coord_dtype=float64), affine=array([[ 2., 0., 0., 0., 0.], [ 0., 3., 0., 0., 0.], [ 0., 0., 4., 0., 0.], [ 0., 0., 0., 5., 0.], [ 0., 0., 0., 0., 1.]]) ) >>> xyz_affine(cmap) array([[ 2., 0., 0., 0.], [ 0., 3., 0., 0.], [ 0., 0., 4., 0.], [ 0., 0., 0., 1.]])
- nipy.core.reference.spaces.xyz_order(coordsys, name2xyz=None)¶
Vector of orders for sorting coordsys axes in xyz first order
- Parameters:
- coordsys
CoordinateSystem
instance - name2xyzNone or mapping, optional
Object such that
name2xyz[ax_name]
returns ‘x’, or ‘y’ or ‘z’ or raises a KeyError for a strax_name
. None means use module default.
- coordsys
- Returns:
- xyz_orderlist
Ordering of axes to get xyz first ordering. See the examples.
- Raises:
- AxesErrorif there are not all of x, y and z axes
Examples
>>> from nipy.core.api import CoordinateSystem >>> xyzt_cs = mni_csm(4) # coordsys with t (time) last >>> xyzt_cs CoordinateSystem(coord_names=('mni-x=L->R', 'mni-y=P->A', 'mni-z=I->S', 't'), name='mni', coord_dtype=float64) >>> xyz_order(xyzt_cs) [0, 1, 2, 3] >>> tzyx_cs = CoordinateSystem(xyzt_cs.coord_names[::-1], 'reversed') >>> tzyx_cs CoordinateSystem(coord_names=('t', 'mni-z=I->S', 'mni-y=P->A', 'mni-x=L->R'), name='reversed', coord_dtype=float64) >>> xyz_order(tzyx_cs) [3, 2, 1, 0]