modalities.fmri.fmristat.model¶
Module: modalities.fmri.fmristat.model
¶
Inheritance diagram for nipy.modalities.fmri.fmristat.model
:
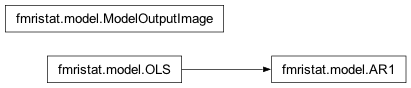
This module defines the two default GLM passes of fmristat
The results of both passes of the GLM get pushed around by generators, which know how to get out the (probably 3D) data for each slice, or parcel (for the AR) case, estimate in 2D, then store the data back again in its original shape.
The containers here, in the execute methods, know how to reshape the data on the way into the estimation (to 2D), then back again, to 3D, or 4D.
It’s relatively easy to do this when just iterating over simple slices, but it gets a bit more complicated when taking arbitrary shaped samples from the image, as we do for estimating the AR coefficients, where we take all the voxels with similar AR coefficients at once.
Classes¶
AR1
¶
- class nipy.modalities.fmri.fmristat.model.AR1(fmri_image, formula, rho, outputs=None, volume_start_times=None)¶
Bases:
OLS
Second pass through fmri_image.
- Parameters:
- fmri_imageFmriImageList
object returning 4D array from
np.asarray
, having attributevolume_start_times
(if volume_start_times is None), and such thatobject[0]
returns something with attributesshape
- formula
nipy.algorithms.statistics.formula.Formula
- rho
Image
image of AR(1) coefficients. Returning data from
rho.get_fdata()
, and having attributecoordmap
- outputslist
Store for model outputs.
- volume_start_times: None or float or (N,) ndarray
start time of each frame. It can be specified either as an ndarray with
N=len(images)
elements or as a single float, the TR. None results innp.arange(len(images)).astype(np.float64)
- Raises:
- ValueError
If volume_start_times not specified, and 4D image passed.
- __init__(fmri_image, formula, rho, outputs=None, volume_start_times=None)¶
- execute()¶
ModelOutputImage
¶
- class nipy.modalities.fmri.fmristat.model.ModelOutputImage(filename, coordmap, shape, clobber=False)¶
Bases:
object
These images have their values filled in as the model is fit, and are saved to disk after being completely filled in.
They are saved to disk by calling the ‘save’ method.
The __getitem__ and __setitem__ calls are delegated to a private Image. An exception is raised if trying to get/set data after the data has been saved to disk.
- __init__(filename, coordmap, shape, clobber=False)¶
- save()¶
Save current Image data to disk
OLS
¶
- class nipy.modalities.fmri.fmristat.model.OLS(fmri_image, formula, outputs=None, volume_start_times=None)¶
Bases:
object
First pass through fmri_image.
- Parameters:
- fmri_imageFmriImageList or 4D image
object returning 4D data from np.asarray, with first (
object[0]
) axis being the independent variable of the model; object[0] returns an object with attributeshape
.- formula
nipy.algorithms.statistics.formula.Formula
- outputslist
Store for model outputs.
- volume_start_times: None or float or (N,) ndarray
start time of each frame. It can be specified either as an ndarray with
N=len(images)
elements or as a single float, the TR. None results innp.arange(len(images)).astype(np.float64)
- Raises:
- ValueError
If volume_start_times not specified, and 4D image passed.
- __init__(fmri_image, formula, outputs=None, volume_start_times=None)¶
- execute()¶
Functions¶
- nipy.modalities.fmri.fmristat.model.estimateAR(resid, design, order=1)¶
Estimate AR parameters using bias correction from fMRIstat.
- Parameters:
- resid: array-like
residuals from model
- model: an OLS model used to estimate residuals
- Returns:
- outputarray
shape (order, resid
- nipy.modalities.fmri.fmristat.model.generate_output(outputs, iterable, reshape=<function <lambda>>)¶
Write out results of a given output.
In the regression setting, results is generally going to be a scipy.stats.models.model.LikelihoodModelResults instance.
- Parameters:
- outputssequence
sequence of output objects
- iterableobject
Object which iterates, returning tuples of (indexer, results), where
indexer
can be used to index into the outputs- reshapecallable
accepts two arguments, first is the indexer, and the second is the array which will be indexed; returns modified indexer and array ready for slicing with modified indexer.
- nipy.modalities.fmri.fmristat.model.model_generator(formula, data, volume_start_times, iterable=None, slicetimes=None, model_type=<class 'nipy.algorithms.statistics.models.regression.OLSModel'>, model_params=<function <lambda>>)¶
Generator for the models for a pass of fmristat analysis.
- nipy.modalities.fmri.fmristat.model.output_AR1(outfile, fmri_image, clobber=False)¶
Create an output file of the AR1 parameter from the OLS pass of fmristat.
- Parameters:
- outfile
- fmri_image
FmriImageList
or 4D image object such that
object[0]
has attributescoordmap
andshape
- clobberbool
if True, overwrite previous output
- Returns:
- regression_output
RegressionOutput
instance
- regression_output
- nipy.modalities.fmri.fmristat.model.output_F(outfile, contrast, fmri_image, clobber=False)¶
output F statistic images
- Parameters:
- outfilestr
filename for F contrast image
- contrastarray
F contrast matrix
- fmri_image
FmriImageList
orImage
object such that
object[0]
has attributesshape
andcoordmap
- clobberbool
if True, overwrites previous output; if False, raises error
- Returns:
- f_reg_out
RegressionOutput
instance Object that can a) be called with a results instance as argument, returning an array, and b) accept the output array for storing, via
obj[slice_spec] = arr
type slicing.
- f_reg_out
- nipy.modalities.fmri.fmristat.model.output_T(outbase, contrast, fmri_image, effect=True, sd=True, t=True, clobber=False)¶
Return t contrast regression outputs list for contrast
- Parameters:
- outbasestring
Base filename that will be used to construct a set of files for the TContrast. For example, outbase=’output.nii’ will result in the following files (assuming defaults for all other params): output_effect.nii, output_sd.nii, output_t.nii
- contrastarray
F contrast matrix
- fmri_image
FmriImageList
orImage
object such that
object[0]
has attributesshape
andcoordmap
- effect{True, False}, optional
whether to write an effect image
- sd{True, False}, optional
whether to write a standard deviation image
- t{True, False}, optional
whether to write a t image
- clobber{False, True}, optional
whether to overwrite images that exist.
- Returns:
- reglist
RegressionOutputList
instance Regression output list with selected outputs, where selection is by inputs effect, sd and t
- reglist
Notes
Note that this routine uses the corresponding
output_T
routine inoutputters
, but indirectly via the TOutput object.
- nipy.modalities.fmri.fmristat.model.output_resid(outfile, fmri_image, clobber=False)¶
Create an output file of the residuals parameter from the OLS pass of fmristat.
Uses affine part of the first image to output resids unless fmri_image is an Image.
- Parameters:
- outfile
- fmri_image
FmriImageList
or 4D image If
FmriImageList
, needs attributesvolume_start_times
, supports len(), and object[0] has attributesaffine
,coordmap
andshape
, from which we create a new 4D coordmap and shape If 4D image, use the images coordmap and shape- clobberbool
if True, overwrite previous output
- Returns:
- regression_output
- nipy.modalities.fmri.fmristat.model.results_generator(model_iterable)¶
Generator for results from an iterator that returns (index, data, model) tuples.
See model_generator.