Generating simulated activation maps¶
The module nipy.labs.utils.simul_multisubject_fmri_dataset
contains a various functions to create simulated activation maps in two, three
and four dimensions. A 2D example is surrogate_2d_dataset()
. The
functions can position various activations and add noise, both as background
noise and jitter in the activation positions and amplitude.
These functions can be useful to test methods.
Example¶
# emacs: -*- mode: python; py-indent-offset: 4; indent-tabs-mode: nil -*-
# vi: set ft=python sts=4 ts=4 sw=4 et:
import numpy as np
import pylab as pl
from nipy.labs.utils.simul_multisubject_fmri_dataset import surrogate_2d_dataset
pos = np.array([[10, 10],
[14, 20],
[23, 18]])
ampli = np.array([4, 5, 2])
# First generate some noiseless data
noiseless_data = surrogate_2d_dataset(n_subj=1, noise_level=0, spatial_jitter=0,
signal_jitter=0, pos=pos, ampli=ampli)
pl.figure(figsize=(10, 3))
pl.subplot(1, 4, 1)
pl.imshow(noiseless_data[0])
pl.title('Noise-less data')
# Second, generate some group data, with default noise parameters
group_data = surrogate_2d_dataset(n_subj=3, pos=pos, ampli=ampli)
pl.subplot(1, 4, 2)
pl.imshow(group_data[0])
pl.title('Subject 1')
pl.subplot(1, 4, 3)
pl.title('Subject 2')
pl.imshow(group_data[1])
pl.subplot(1, 4, 4)
pl.title('Subject 3')
pl.imshow(group_data[2])
(Source code
, png
, hires.png
, pdf
)
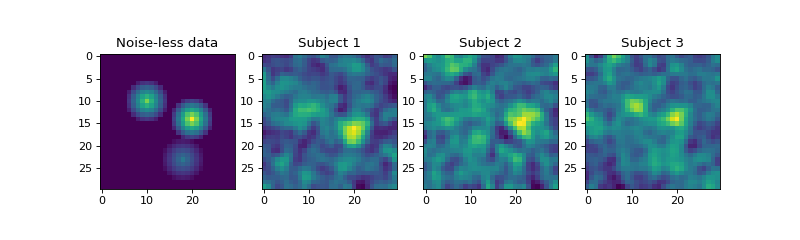
Function documentation¶
- nipy.labs.utils.simul_multisubject_fmri_dataset.surrogate_2d_dataset(n_subj=10, shape=(30, 30), sk=1.0, noise_level=1.0, pos=array([[6, 7], [10, 10], [15, 10]]), ampli=array([3, 4, 4]), spatial_jitter=1.0, signal_jitter=1.0, width=5.0, width_jitter=0, out_text_file=None, out_image_file=None, seed=False)¶
Create surrogate (simulated) 2D activation data with spatial noise
- Parameters:
- n_subj: integer, optional
The number of subjects, ie the number of different maps generated.
- shape=(30,30): tuple of integers,
the shape of each image
- sk: float, optional
Amount of spatial noise smoothness.
- noise_level: float, optional
Amplitude of the spatial noise. amplitude=noise_level)
- pos: 2D ndarray of integers, optional
x, y positions of the various simulated activations.
- ampli: 1D ndarray of floats, optional
Respective amplitude of each activation
- spatial_jitter: float, optional
Random spatial jitter added to the position of each activation, in pixel.
- signal_jitter: float, optional
Random amplitude fluctuation for each activation, added to the amplitude specified by ampli
- width: float or ndarray, optional
Width of the activations
- width_jitter: float
Relative width jitter of the blobs
- out_text_file: string or None, optional
If not None, the resulting array is saved as a text file with the given file name
- out_image_file: string or None, optional
If not None, the resulting is saved as a nifti file with the given file name.
- seed=False: int, optional
If seed is not False, the random number generator is initialized at a certain value
- Returns:
- dataset: 3D ndarray
The surrogate activation map, with dimensions
(n_subj,) + shape
- nipy.labs.utils.simul_multisubject_fmri_dataset.surrogate_3d_dataset(n_subj=1, shape=(20, 20, 20), mask=None, sk=1.0, noise_level=1.0, pos=None, ampli=None, spatial_jitter=1.0, signal_jitter=1.0, width=5.0, out_text_file=None, out_image_file=None, seed=False)¶
Create surrogate (simulated) 3D activation data with spatial noise.
- Parameters:
- n_subj: integer, optional
The number of subjects, ie the number of different maps generated.
- shape=(20,20,20): tuple of 3 integers,
the shape of each image
- mask=None: Nifti1Image instance,
referential- and mask- defining image (overrides shape)
- sk: float, optional
Amount of spatial noise smoothness.
- noise_level: float, optional
Amplitude of the spatial noise. amplitude=noise_level)
- pos: 2D ndarray of integers, optional
x, y positions of the various simulated activations.
- ampli: 1D ndarray of floats, optional
Respective amplitude of each activation
- spatial_jitter: float, optional
Random spatial jitter added to the position of each activation, in pixel.
- signal_jitter: float, optional
Random amplitude fluctuation for each activation, added to the amplitude specified by ampli
- width: float or ndarray, optional
Width of the activations
- out_text_file: string or None, optional
If not None, the resulting array is saved as a text file with the given file name
- out_image_file: string or None, optional
If not None, the resulting is saved as a nifti file with the given file name.
- seed=False: int, optional
If seed is not False, the random number generator is initialized at a certain value
- Returns:
- dataset: 3D ndarray
The surrogate activation map, with dimensions
(n_subj,) + shape
- nipy.labs.utils.simul_multisubject_fmri_dataset.surrogate_4d_dataset(shape=(20, 20, 20), mask=None, n_scans=1, n_sess=1, dmtx=None, sk=1.0, noise_level=1.0, signal_level=1.0, out_image_file=None, seed=False)¶
Create surrogate (simulated) 3D activation data with spatial noise.
- Parameters:
- shape = (20, 20, 20): tuple of integers,
the shape of each image
- mask=None: brifti image instance,
referential- and mask- defining image (overrides shape)
- n_scans: int, optional,
number of scans to be simlulated overridden by the design matrix
- n_sess: int, optional,
the number of simulated sessions
- dmtx: array of shape(n_scans, n_rows),
the design matrix
- sk: float, optional
Amount of spatial noise smoothness.
- noise_level: float, optional
Amplitude of the spatial noise. amplitude=noise_level)
- signal_level: float, optional,
Amplitude of the signal
- out_image_file: string or list of strings or None, optional
If not None, the resulting is saved as (set of) nifti file(s) with the given file path(s)
- seed=False: int, optional
If seed is not False, the random number generator is initialized at a certain value
- Returns:
- dataset: a list of n_sess ndarray of shape
(shape[0], shape[1], shape[2], n_scans) The surrogate activation map