algorithms.statistics.rft¶
Module: algorithms.statistics.rft
¶
Inheritance diagram for nipy.algorithms.statistics.rft
:
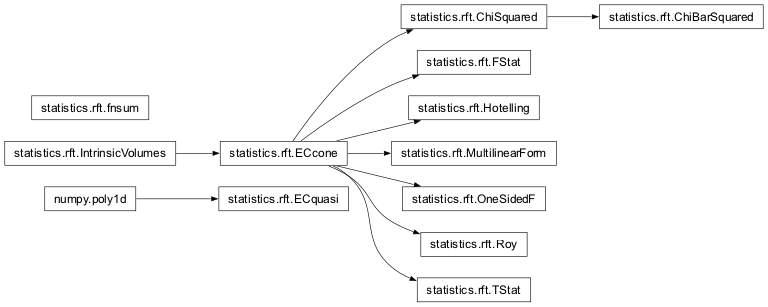
Random field theory routines
The theoretical results for the EC densities appearing in this module were partially supported by NSF grant DMS-0405970.
- Taylor, J.E. & Worsley, K.J. (2012). “Detecting sparse cone alternatives
for Gaussian random fields, with an application to fMRI”. arXiv:1207.3840 [math.ST] and Statistica Sinica 23 (2013): 1629-1656.
- Taylor, J.E. & Worsley, K.J. (2008). “Random fields of multivariate
test statistics, with applications to shape analysis.” arXiv:0803.1708 [math.ST] and Annals of Statistics 36( 2008): 1-27
Classes¶
ChiBarSquared
¶
- class nipy.algorithms.statistics.rft.ChiBarSquared(dfn=1, search=[1])¶
Bases:
ChiSquared
- __init__(dfn=1, search=[1])¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
ChiSquared
¶
- class nipy.algorithms.statistics.rft.ChiSquared(dfn, dfd=inf, search=[1])¶
Bases:
ECcone
EC densities for a Chi-Squared(n) random field.
- __init__(dfn, dfd=inf, search=[1])¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
ECcone
¶
- class nipy.algorithms.statistics.rft.ECcone(mu=[1], dfd=inf, search=[1], product=[1])¶
Bases:
IntrinsicVolumes
EC approximation to supremum distribution of var==1 Gaussian process
A class that takes the intrinsic volumes of a set and gives the EC approximation to the supremum distribution of a unit variance Gaussian process with these intrinsic volumes. This is the basic building block of all of the EC densities.
If product is not None, then this product (an instance of IntrinsicVolumes) will effectively be prepended to the search region in any call, but it will also affect the (quasi-)polynomial part of the EC density. For instance, Hotelling’s T^2 random field has a sphere as product, as does Roy’s maximum root.
- __init__(mu=[1], dfd=inf, search=[1], product=[1])¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
ECquasi
¶
- class nipy.algorithms.statistics.rft.ECquasi(c_or_r, r=0, exponent=None, m=None)¶
Bases:
poly1d
Polynomials with premultiplier
A subclass of poly1d consisting of polynomials with a premultiplier of the form:
(1 + x^2/m)^-exponent
where m is a non-negative float (possibly infinity, in which case the function is a polynomial) and exponent is a non-negative multiple of 1/2.
These arise often in the EC densities.
Examples
>>> import numpy >>> from nipy.algorithms.statistics.rft import ECquasi >>> x = numpy.linspace(0,1,101)
>>> a = ECquasi([3,4,5]) >>> a ECquasi(array([3, 4, 5]), m=inf, exponent=0.000000) >>> a(3) == 3*3**2 + 4*3 + 5 True
>>> b = ECquasi(a.coeffs, m=30, exponent=4) >>> numpy.allclose(b(x), a(x) * numpy.power(1+x**2/30, -4)) True
- __init__(c_or_r, r=0, exponent=None, m=None)¶
- property c¶
The polynomial coefficients
- change_exponent(_pow)¶
Change exponent
Multiply top and bottom by an integer multiple of the self.denom_poly.
Examples
>>> import numpy >>> b = ECquasi([3,4,20], m=30, exponent=4) >>> x = numpy.linspace(0,1,101) >>> c = b.change_exponent(3) >>> c ECquasi(array([ 1.11111111e-04, 1.48148148e-04, 1.07407407e-02, 1.33333333e-02, 3.66666667e-01, 4.00000000e-01, 5.00000000e+00, 4.00000000e+00, 2.00000000e+01]), m=30.000000, exponent=7.000000) >>> numpy.allclose(c(x), b(x)) True
- property coef¶
The polynomial coefficients
- property coefficients¶
The polynomial coefficients
- property coeffs¶
The polynomial coefficients
- compatible(other)¶
Check compatibility of degrees of freedom
Check whether the degrees of freedom of two instances are equal so that they can be multiplied together.
Examples
>>> import numpy >>> b = ECquasi([3,4,20], m=30, exponent=4) >>> x = numpy.linspace(0,1,101) >>> c = b.change_exponent(3) >>> b.compatible(c) True >>> d = ECquasi([3,4,20]) >>> b.compatible(d) False >>>
- denom_poly()¶
Base of the premultiplier: (1+x^2/m).
Examples
>>> import numpy >>> b = ECquasi([3,4,20], m=30, exponent=4) >>> d = b.denom_poly() >>> d poly1d([ 0.03333333, 0. , 1. ]) >>> numpy.allclose(d.c, [1./b.m,0,1]) True
- deriv(m=1)¶
Evaluate derivative of ECquasi
- Parameters:
- mint, optional
Examples
>>> a = ECquasi([3,4,5]) >>> a.deriv(m=2) ECquasi(array([6]), m=inf, exponent=0.000000)
>>> b = ECquasi([3,4,5], m=10, exponent=3) >>> b.deriv() ECquasi(array([-1.2, -2. , 3. , 4. ]), m=10.000000, exponent=4.000000)
- integ(m=1, k=0)¶
Return an antiderivative (indefinite integral) of this polynomial.
Refer to polyint for full documentation.
See also
polyint
equivalent function
- property o¶
The order or degree of the polynomial
- property order¶
The order or degree of the polynomial
- property r¶
The roots of the polynomial, where self(x) == 0
- property roots¶
The roots of the polynomial, where self(x) == 0
- property variable¶
The name of the polynomial variable
FStat
¶
- class nipy.algorithms.statistics.rft.FStat(dfn, dfd=inf, search=[1])¶
Bases:
ECcone
EC densities for a F random field.
- __init__(dfn, dfd=inf, search=[1])¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
Hotelling
¶
- class nipy.algorithms.statistics.rft.Hotelling(dfd=inf, k=1, search=[1])¶
Bases:
ECcone
Hotelling’s T^2
Maximize an F_{1,dfd}=T_dfd^2 statistic over a sphere of dimension k.
- __init__(dfd=inf, k=1, search=[1])¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
IntrinsicVolumes
¶
MultilinearForm
¶
- class nipy.algorithms.statistics.rft.MultilinearForm(*dims, **keywords)¶
Bases:
ECcone
Maximize a multivariate Gaussian form
Maximized over spheres of dimension dims. See:
Kuri, S. & Takemura, A. (2001). ‘Tail probabilities of the maxima of multilinear forms and their applications.’ Ann. Statist. 29(2): 328-371.
- __init__(*dims, **keywords)¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
OneSidedF
¶
- class nipy.algorithms.statistics.rft.OneSidedF(dfn, dfd=inf, search=[1])¶
Bases:
ECcone
EC densities for one-sided F statistic
See:
Worsley, K.J. & Taylor, J.E. (2005). ‘Detecting fMRI activation allowing for unknown latency of the hemodynamic response.’ Neuroimage, 29,649-654.
- __init__(dfn, dfd=inf, search=[1])¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
Roy
¶
- class nipy.algorithms.statistics.rft.Roy(dfn=1, dfd=inf, k=1, search=[1])¶
Bases:
ECcone
Roy’s maximum root
Maximize an F_{dfd,dfn} statistic over a sphere of dimension k.
- __init__(dfn=1, dfd=inf, k=1, search=[1])¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
TStat
¶
- class nipy.algorithms.statistics.rft.TStat(dfd=inf, search=[1])¶
Bases:
ECcone
EC densities for a t random field.
- __init__(dfd=inf, search=[1])¶
- density(x, dim)¶
The EC density in dimension dim.
- integ(m=None, k=None)¶
- pvalue(x, search=None)¶
- quasi(dim)¶
(Quasi-)polynomial parts of EC density in dimension dim
ignoring a factor of (2pi)^{-(dim+1)/2} in front.
fnsum
¶
Functions¶
- nipy.algorithms.statistics.rft.Q(dim, dfd=inf)¶
Q polynomial
If dfd == inf (the default), then Q(dim) is the (dim-1)-st Hermite polynomial:
\[H_j(x) = (-1)^j * e^{x^2/2} * (d^j/dx^j e^{-x^2/2})\]If dfd != inf, then it is the polynomial Q defined in [Worsley1994]
- Parameters:
- dimint
dimension of polynomial
- dfdscalar
- Returns:
- q_polynp.poly1d instance
References
[Worsley1994]Worsley, K.J. (1994). ‘Local maxima and the expected Euler characteristic of excursion sets of chi^2, F and t fields.’ Advances in Applied Probability, 26:13-42.
- nipy.algorithms.statistics.rft.ball_search(n, r=1)¶
A ball-shaped search region of radius r.
- nipy.algorithms.statistics.rft.binomial(n, k)¶
Binomial coefficient
n!
- c = ———
(n-k)! k!
- Parameters:
- nfloat
n of (n, k)
- kfloat
k of (n, k)
- Returns:
- cfloat
Examples
First 3 values of 4 th row of Pascal triangle
>>> [binomial(4, k) for k in range(3)] [1.0, 4.0, 6.0]
- nipy.algorithms.statistics.rft.mu_ball(n, j, r=1)¶
j`th curvature of `n-dimensional ball radius r
Return mu_j(B_n(r)), the j-th Lipschitz Killing curvature of the ball of radius r in R^n.
- nipy.algorithms.statistics.rft.mu_sphere(n, j, r=1)¶
j`th curvature for `n dimensional sphere radius r
Return mu_j(S_r(R^n)), the j-th Lipschitz Killing curvature of the sphere of radius r in R^n.
From Chapter 6 of
Adler & Taylor, ‘Random Fields and Geometry’. 2006.
- nipy.algorithms.statistics.rft.scale_space(region, interval, kappa=1.0)¶
scale space intrinsic volumes of region x interval
See:
Siegmund, D.O and Worsley, K.J. (1995). ‘Testing for a signal with unknown location and scale in a stationary Gaussian random field.’ Annals of Statistics, 23:608-639.
and
Taylor, J.E. & Worsley, K.J. (2005). ‘Random fields of multivariate test statistics, with applications to shape analysis and fMRI.’
(available on http://www.math.mcgill.ca/keith
- nipy.algorithms.statistics.rft.spherical_search(n, r=1)¶
A spherical search region of radius r.
- nipy.algorithms.statistics.rft.volume2ball(vol, d=3)¶
Approximate volume with ball
Approximate intrinsic volumes of a set with a given volume by those of a ball with a given dimension and equal volume.