core.reference.array_coords¶
Module: core.reference.array_coords
¶
Inheritance diagram for nipy.core.reference.array_coords
:
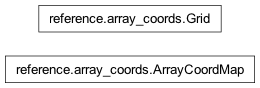
Some CoordinateMaps have a domain that are ‘array’ coordinates, hence the function of the CoordinateMap can be evaluated at these ‘array’ points.
This module tries to make these operations easier by defining a class ArrayCoordMap that is essentially a CoordinateMap and a shape.
This class has two properties: values, transposed_values the CoordinateMap at np.indices(shape).
The class Grid is meant to take a CoordinateMap and an np.mgrid-like notation to create an ArrayCoordMap.
Classes¶
ArrayCoordMap
¶
- class nipy.core.reference.array_coords.ArrayCoordMap(coordmap, shape)¶
Bases:
object
Class combining coordinate map and array shape
When the function_domain of a CoordinateMap can be thought of as ‘array’ coordinates, i.e. an ‘input_shape’ makes sense. We can than evaluate the CoordinateMap at np.indices(input_shape)
- __init__(coordmap, shape)¶
- Parameters:
- coordmap
CoordinateMap
A CoordinateMap with function_domain that are ‘array’ coordinates.
- shapesequence of int
The size of the (implied) underlying array.
- coordmap
Examples
>>> aff = np.diag([0.6,1.1,2.3,1]) >>> aff[:3,3] = (0.1, 0.2, 0.3) >>> cmap = AffineTransform.from_params('ijk', 'xyz', aff) >>> cmap.ndims # number of (input, output) dimensions (3, 3) >>> acmap = ArrayCoordMap(cmap, (1, 2, 3))
Real world values at each array coordinate, one row per array coordinate (6 in this case), one column for each output dimension (3 in this case)
>>> acmap.values array([[ 0.1, 0.2, 0.3], [ 0.1, 0.2, 2.6], [ 0.1, 0.2, 4.9], [ 0.1, 1.3, 0.3], [ 0.1, 1.3, 2.6], [ 0.1, 1.3, 4.9]])
Same values, but arranged in np.indices / np.mgrid format, first axis is for number of output coordinates (3 in our case), the rest are for the input shape (1, 2, 3)
>>> acmap.transposed_values.shape (3, 1, 2, 3) >>> acmap.transposed_values array([[[[ 0.1, 0.1, 0.1], [ 0.1, 0.1, 0.1]]], [[[ 0.2, 0.2, 0.2], [ 1.3, 1.3, 1.3]]], [[[ 0.3, 2.6, 4.9], [ 0.3, 2.6, 4.9]]]])
- static from_shape(coordmap, shape)¶
Create an evaluator assuming that coordmap.function_domain are ‘array’ coordinates.
- property transposed_values¶
Get values of ArrayCoordMap in an array of shape (self.coordmap.ndims[1],) + self.shape)
- property values¶
Get values of ArrayCoordMap in a 2-dimensional array of shape (product(self.shape), self.coordmap.ndims[1]))
Grid
¶
- class nipy.core.reference.array_coords.Grid(coords)¶
Bases:
object
Simple class to construct AffineTransform instances with slice notation like np.ogrid/np.mgrid.
>>> c = CoordinateSystem('xy', 'input') >>> g = Grid(c) >>> points = g[-1:1:21j,-2:4:31j] >>> points.coordmap.affine array([[ 0.1, 0. , -1. ], [ 0. , 0.2, -2. ], [ 0. , 0. , 1. ]])
>>> print(points.coordmap.function_domain) CoordinateSystem(coord_names=('i0', 'i1'), name='product', coord_dtype=float64) >>> print(points.coordmap.function_range) CoordinateSystem(coord_names=('x', 'y'), name='input', coord_dtype=float64)
>>> points.shape (21, 31) >>> print(points.transposed_values.shape) (2, 21, 31) >>> print(points.values.shape) (651, 2)
- __init__(coords)¶
Initialize Grid object
- Parameters:
- coords: ``CoordinateMap`` or ``CoordinateSystem``
A coordinate map to be ‘sliced’ into. If coords is a CoordinateSystem, then an AffineTransform instance is created with coords with identity transformation.