core.reference.coordinate_system¶
Module: core.reference.coordinate_system
¶
Inheritance diagram for nipy.core.reference.coordinate_system
:
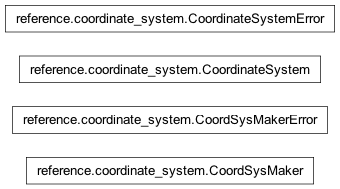
CoordinateSystems are used to represent the space in which the image resides.
A CoordinateSystem contains named coordinates, one for each dimension and a coordinate dtype. The purpose of the CoordinateSystem is to specify the name and order of the coordinate axes for a particular space. This allows one to compare two CoordinateSystems to determine if they are equal.
Classes¶
CoordSysMaker
¶
- class nipy.core.reference.coordinate_system.CoordSysMaker(coord_names, name='', coord_dtype=<class 'numpy.float64'>)¶
Bases:
object
Class to create similar coordinate maps of different dimensions
- __init__(coord_names, name='', coord_dtype=<class 'numpy.float64'>)¶
Create a coordsys maker with given axis coord_names
- Parameters:
- coord_namesiterable
A sequence of coordinate names.
- namestring, optional
The name of the coordinate system
- coord_dtypenp.dtype, optional
The dtype of the coord_names. This should be a built-in numpy scalar dtype. (default is np.float64). The value can by anything that can be passed to the np.dtype constructor. For example
np.float64
,np.dtype(np.float64)
orf8
all result in the samecoord_dtype
.
Examples
>>> cmkr = CoordSysMaker('ijk', 'a name') >>> print(cmkr(2)) CoordinateSystem(coord_names=('i', 'j'), name='a name', coord_dtype=float64) >>> print(cmkr(3)) CoordinateSystem(coord_names=('i', 'j', 'k'), name='a name', coord_dtype=float64)
- coord_sys_klass¶
alias of
CoordinateSystem
CoordSysMakerError
¶
CoordinateSystem
¶
- class nipy.core.reference.coordinate_system.CoordinateSystem(coord_names, name='', coord_dtype=<class 'numpy.float64'>)¶
Bases:
object
An ordered sequence of named coordinates of a specified dtype.
A coordinate system is defined by the names of the coordinates, (attribute
coord_names
) and the numpy dtype of each coordinate value (attributecoord_dtype
). The coordinate system can also have a name.>>> names = ['first', 'second', 'third'] >>> cs = CoordinateSystem(names, 'a coordinate system', np.float64) >>> cs.coord_names ('first', 'second', 'third') >>> cs.name 'a coordinate system' >>> cs.coord_dtype dtype('float64')
The coordinate system also has a
dtype
which is the composite numpy dtype, made from the (names
,coord_dtype
).>>> dtype_template = [(name, np.float64) for name in cs.coord_names] >>> dtype_should_be = np.dtype(dtype_template) >>> cs.dtype == dtype_should_be True
Two CoordinateSystems are equal if they have the same dtype and the same names and the same name.
>>> another_cs = CoordinateSystem(names, 'not irrelevant', np.float64) >>> cs == another_cs False >>> cs.dtype == another_cs.dtype True >>> cs.name == another_cs.name False
- __init__(coord_names, name='', coord_dtype=<class 'numpy.float64'>)¶
Create a coordinate system with a given name and coordinate names.
The CoordinateSystem has two dtype attributes:
self.coord_dtype is the dtype of the individual coordinate values
self.dtype is the recarray dtype for the CoordinateSystem which combines the coord_names and the coord_dtype. This functions as the description of the CoordinateSystem.
- Parameters:
- coord_namesiterable
A sequence of coordinate names.
- namestring, optional
The name of the coordinate system
- coord_dtypenp.dtype, optional
The dtype of the coord_names. This should be a built-in numpy scalar dtype. (default is np.float64). The value can by anything that can be passed to the np.dtype constructor. For example
np.float64
,np.dtype(np.float64)
orf8
all result in the samecoord_dtype
.
Examples
>>> c = CoordinateSystem('ij', name='input') >>> print(c) CoordinateSystem(coord_names=('i', 'j'), name='input', coord_dtype=float64) >>> c.coord_dtype dtype('float64')
- coord_dtype¶
alias of
float64
- coord_names = ('x', 'y', 'z')¶
- dtype = dtype([('x', '<f8'), ('y', '<f8'), ('z', '<f8')])¶
- index(coord_name)¶
Return the index of a given named coordinate.
>>> c = CoordinateSystem('ij', name='input') >>> c.index('i') 0 >>> c.index('j') 1
- name = 'world-LPI'¶
- ndim = 3¶
- similar_to(other)¶
Similarity is defined by self.dtype, ignoring name
- Parameters:
- other
CoordinateSystem
The object to be compared with
- other
- Returns:
- tf: bool
CoordinateSystemError
¶
Functions¶
- nipy.core.reference.coordinate_system.is_coordsys(obj)¶
Test if obj has the CoordinateSystem API
- Parameters:
- objobject
Object to test
- Returns:
- tfbool
True if obj has the coordinate system API
Examples
>>> is_coordsys(CoordinateSystem('xyz')) True >>> is_coordsys(CoordSysMaker('ikj')) False
- nipy.core.reference.coordinate_system.is_coordsys_maker(obj)¶
Test if obj has the CoordSysMaker API
- Parameters:
- objobject
Object to test
- Returns:
- tfbool
True if obj has the coordinate system API
Examples
>>> is_coordsys_maker(CoordSysMaker('ikj')) True >>> is_coordsys_maker(CoordinateSystem('xyz')) False
- nipy.core.reference.coordinate_system.product(*coord_systems, **kwargs)¶
Create the product of a sequence of CoordinateSystems.
The coord_dtype of the result will be determined by
safe_dtype
.- Parameters:
- *coord_systemssequence of
CoordinateSystem
- namestr
Name of output coordinate system
- *coord_systemssequence of
- Returns:
- product_coord_system
CoordinateSystem
- product_coord_system
Examples
>>> c1 = CoordinateSystem('ij', 'input', coord_dtype=np.float32) >>> c2 = CoordinateSystem('kl', 'input', coord_dtype=np.complex_) >>> c3 = CoordinateSystem('ik', 'in3')
>>> print(product(c1, c2)) CoordinateSystem(coord_names=('i', 'j', 'k', 'l'), name='product', coord_dtype=complex128)
>>> print(product(c1, c2, name='another name')) CoordinateSystem(coord_names=('i', 'j', 'k', 'l'), name='another name', coord_dtype=complex128)
>>> product(c2, c3) Traceback (most recent call last): ... ValueError: coord_names must have distinct names
- nipy.core.reference.coordinate_system.safe_dtype(*dtypes)¶
Determine a dtype to safely cast all of the given dtypes to.
Safe dtypes are valid numpy dtypes or python types which can be cast to numpy dtypes. See numpy.sctypes for a list of valid dtypes. Composite dtypes and string dtypes are not safe dtypes.
- Parameters:
- dtypessequence of
np.dtype
- dtypessequence of
- Returns:
- dtypenp.dtype
Examples
>>> c1 = CoordinateSystem('ij', 'input', coord_dtype=np.float32) >>> c2 = CoordinateSystem('kl', 'input', coord_dtype=np.complex_) >>> safe_dtype(c1.coord_dtype, c2.coord_dtype) dtype('complex128')
>>> # Strings are invalid dtypes >>> safe_dtype(type('foo')) Traceback (most recent call last): ... TypeError: dtype must be valid numpy dtype int, uint, float, complex or object
>>> # Check for a valid dtype >>> myarr = np.zeros(2, np.float32) >>> myarr.dtype.isbuiltin 1
>>> # Composite dtypes are invalid >>> mydtype = np.dtype([('name', 'S32'), ('age', 'i4')]) >>> myarr = np.zeros(2, mydtype) >>> myarr.dtype.isbuiltin 0 >>> safe_dtype(mydtype) Traceback (most recent call last): ... TypeError: dtype must be valid numpy dtype int, uint, float, complex or object