algorithms.statistics.models.utils¶
Module: algorithms.statistics.models.utils
¶
Inheritance diagram for nipy.algorithms.statistics.models.utils
:
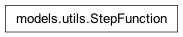
General matrix and other utilities for statistics
Class¶
StepFunction
¶
- class nipy.algorithms.statistics.models.utils.StepFunction(x, y, ival=0.0, sorted=False)¶
Bases:
object
A basic step function
Values at the ends are handled in the simplest way possible: everything to the left of
x[0]
is set to ival; everything to the right ofx[-1]
is set toy[-1]
.Examples
>>> x = np.arange(20) >>> y = np.arange(20) >>> f = StepFunction(x, y) >>> >>> f(3.2) 3.0 >>> res = f([[3.2, 4.5],[24, -3.1]]) >>> np.all(res == [[ 3, 4], ... [19, 0]]) True
- __init__(x, y, ival=0.0, sorted=False)¶
Functions¶
- nipy.algorithms.statistics.models.utils.ECDF(values)¶
Return the ECDF of an array as a step function.
- nipy.algorithms.statistics.models.utils.mad(a, c=0.6745, axis=0)¶
Median Absolute Deviation:
median(abs(a - median(a))) / c
- nipy.algorithms.statistics.models.utils.monotone_fn_inverter(fn, x, vectorized=True, **keywords)¶
Given a monotone function x (no checking is done to verify monotonicity) and a set of x values, return an linearly interpolated approximation to its inverse from its values on x.